DIY Project: Arduino Mini Piano
For this post, we will be going through the steps in creating a mini piano using an Arduino board. It is basically an audio player, as it set up using pushbuttons which play audio files from the SD card. To accomplish this, we will be using one of the libraries created by the Arduino community to easily enable the Arduino to play the audio from the SD card.
Hardware required
If the Arduino is not able to detect the SD card you will get the error message below:
For this project, we used the 3.3V power supply for the SD card module and the 5V power supply for the breadboard to power the push buttons and the speaker.
The Audio Output Pin for the Arduino is Pin 9, so connect the speaker to Pin 9. The connecting Pins for the push buttons are listed below.
Here's a bird's eye view of the complete circuit on the breadboard.
Programming the Arduino
Once we are done with setting up the circuit hardware, now to programme the code for the Arduino to run. Insert the SD card into the module and follow these steps.
Step 1: We used the TMRpcm library in making the Arduino run the functions for this project. This library enables asynchronous playback of the audio waveform files.
Note: For this project, we chose to use one audio file and have the different push buttons set up to play from different points in the audio file, by adding the time in seconds below to tell Arduino to play from that point (time in the track).
For example:
tunes.play("0.wav",20); //play track from the 20th second
See it in Action
The video below shows the project in action and explains how it works. It is really simple to operate, you push any of the four push buttons and it plays the audio.
Hardware required
- Arduino board (For this project, we are using an Arduino Uno)
- SD card reader module
- SD card (If using a micro SD card, you will also need micro SD card Adapter)
- Speaker
- 220Ω Resistors (5 of them)
- Push buttons (4 of them)
- Jumper Wires
- and a Breadboard for easy connection
Setting Up your Audio Files on your SD card
The Arduino board only recognizes audio files in Waveform format (.wav) so you will need to convert your mp3 files to this format.
Step 1: Go to the Online Wav convert website
The Arduino board only recognizes audio files in Waveform format (.wav) so you will need to convert your mp3 files to this format.
Step 1: Go to the Online Wav convert website
Step 2: Choose and upload your audio file to the website.
Step 3: Choose your conversion settings. Here's a recommendation for the best sound quality for your conversion retrieved from B. Raj's DIY audio player project (a reference to the previous DIY analysis post).
Step 3: Choose your conversion settings. Here's a recommendation for the best sound quality for your conversion retrieved from B. Raj's DIY audio player project (a reference to the previous DIY analysis post).
You can also trim the length of your audio for your conversion, which made it easy for cutting out the bits of audio for this piano.
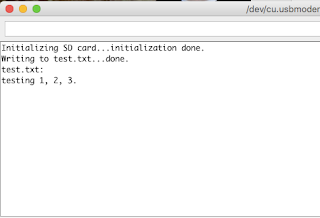
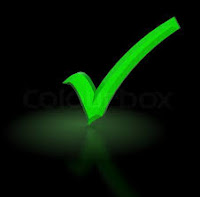
Step 4: After you are satisfied with the settings and trim (if needed), click "Convert file" and the website will convert the file and automatically download it when finished.
Step 5: Now that the audio files are ready. It's time to work on the SD card. You have to format your SD card before using it on the Arduino.
I used Disk Utility on the Macbook. When formatting the SD card, make sure to choose the format as FAT, otherwise, the Arduino will not be able to initialize the SD card.
Make sure to select the MS-DOS (FAT) format for your SD card as the Arduino can only detect SD cards in the FAT format.
After the format the SD card is good to now operate on the Arduino, so save your waveform audio files on the SD card.
Note: You can test your SD card using the examples on the Arduino (after the connecting the SD card to it) to make sure the card is working properly. You can find the examples under File>Examples>SD. Upload the code and open the serial monitor to check.
Here's a snippet using the SD ReadWrite example:
This is what you will see if the card is formatted properly and is working:
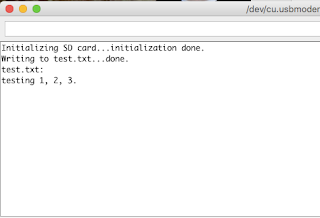
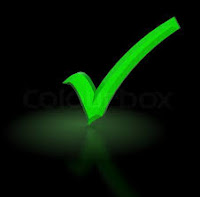
If the Arduino is not able to detect the SD card you will get the error message below:
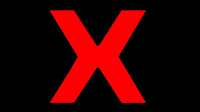
Connecting the Circuit
Connecting the circuit for this project is fairly simple. It is made of 4 pushbutton circuits, the SD card reader module circuit and the speaker circuit, which all connecting to pins on the Arduino.
This is the schematic for the complete circuit connection.
The audio files are stored in the micro SD card, which has been inserted into an adapter and then mounted onto the SD card reader module. The connecting pins for SD card are as follows in the table below.
The Audio Output Pin for the Arduino is Pin 9, so connect the speaker to Pin 9. The connecting Pins for the push buttons are listed below.
Here's a bird's eye view of the complete circuit on the breadboard.
Note: The connecting pins for the SD card reader module and Speaker pin differ depending on the version of your Arduino board. So make sure to check the schematic for your version of the Arduino board to make sure you are connecting to the correct pins. This information can be found on the Arduino site, on the relevant board page under the documentation section. For example, here's the link to the Arduino Uno Rev3 page: https://store.arduino.cc/usa/arduino-uno-rev3
Programming the Arduino
Once we are done with setting up the circuit hardware, now to programme the code for the Arduino to run. Insert the SD card into the module and follow these steps.
Step 1: We used the TMRpcm library in making the Arduino run the functions for this project. This library enables asynchronous playback of the audio waveform files.
- To download the library, click here
- Select "Clone or Download"
- Select "Download Zip"
Step 2: Include the Zip file in your Arduino IDE by selecting the following: Sketch>Include Library>Add ZIP. library...then choose the downloaded zip file from your computer.
With the inclusion of the library in your code, the Arduino will be able to play the audio files.
The Code
The full set of the program code is provided below, with notes explaining the functions of the lines.
/*
Arduino Mini piano
This is a one-handed piano with four keys using push buttons.
The circuit:
* Push Buttons on Pin 2 (blue),3 (green),4 (black) and 5 (white)
* Audio Output (Speaker) - Pin 9
SD card attached to SPI bus (SD card reader module) pins below:
* CS - Pin 10
* MOSI - Pin 11
* MISO - Pin 12
* SCK - Pin 13
created March 24th, 2018
by Nina Oghoore, Kaiyi and Asmaa
*/
#include "SD.h"
#define SD_ChipSelectPin 10
#include "TMRpcm.h"
#include "SPI.h"
TMRpcm tunes;
boolean playingMusic1=false; //can either be true (audio is playing) or false (audio is not playing)
boolean playingMusic2=false; //can either be true (audio is playing) or false (audio is not playing)
boolean playingMusic3=false; //can either be true (audio is playing) or false (audio is not playing)
boolean playingMusic4=false; //can either be true (audio is playing) or false (audio is not playing)
void setup(){
tunes.speakerPin = 9;
Serial.begin(9600);
if (!SD.begin(SD_ChipSelectPin)) {
Serial.println("SD fail");
return;
}
pinMode(2,INPUT); //PushButton1 to play track
pinMode(3,INPUT); //PushButton2 to play track
pinMode(4,INPUT); //PushButton3 to play track
pinMode(5,INPUT); //PushButton4 to play track
pinMode(9,OUTPUT); //Speaker pin
tunes.setVolume(5); //Volume range between 0 and 7
//tunes.quality(0);
}
void loop() {
Push1(); //Pulls and loops function below
Push2(); //Pulls and loops function below
Push3(); //Pulls and loops function below
Push4(); //Pulls and loops function below
}
void Push1() {
if (digitalRead(2)==LOW && playingMusic1==false) //PushButton1 pressed and audio is off
{
playingMusic1=true; //turn on audio
Serial.println("push1 detected"); //print to serial monitor
tunes.play("0.wav",33); //play track from the 33rd second
}
if (digitalRead(2)==HIGH && playingMusic1==true) //PushButton1 not pressed and audio is on
{
tunes.pause(); //turn off audio
playingMusic1=false; //audio is off
}
}
void Push2() {
if (digitalRead(3)==LOW && playingMusic2==false) //PushButton2 pressed and audio is off
{
playingMusic2=true; //turn on audio
Serial.println("push2 detected"); //print to serial monitor
tunes.play("0.wav",120); //play track from the 120th second
}
if (digitalRead(3)==HIGH && playingMusic2==true) //PushButton2 not pressed and audio is on
{
tunes.pause(); //turn off audio
playingMusic2=false; //audio is off
}
}
void Push3() {
if (digitalRead(4)==LOW && playingMusic3==false) //PushButton3 pressed and audio is off
{
playingMusic3=true; //turn on audio
Serial.println("push3 detected"); //print to serial monitor
tunes.play("0.wav",59); //play track from the 59th second
}
if (digitalRead(4)==HIGH && playingMusic3==true) //PushButton3 not pressed and audio is on
{
tunes.pause(); //turn off audio
playingMusic3=false; //audio is off
}
}
void Push4() {
if (digitalRead(5)==LOW && playingMusic4==false) //PushButton4 pressed and audio is off
{
playingMusic4=true; //turn on audio
Serial.println("push4 detected"); //print to serial monitor
tunes.play("0.wav",20); //play track from the 20th second
}
if (digitalRead(5)==HIGH && playingMusic4==true) //PushButton4 not pressed and audio is on
{
tunes.pause(); //turn off audio
playingMusic4=false; //audio is off
}
}
Step 3: Add the TMRpcm library to your sketch by selecting the following: Sketch>Include Library>TMRpcm.
The Code
The full set of the program code is provided below, with notes explaining the functions of the lines.
/*
Arduino Mini piano
This is a one-handed piano with four keys using push buttons.
The circuit:
* Push Buttons on Pin 2 (blue),3 (green),4 (black) and 5 (white)
* Audio Output (Speaker) - Pin 9
SD card attached to SPI bus (SD card reader module) pins below:
* CS - Pin 10
* MOSI - Pin 11
* MISO - Pin 12
* SCK - Pin 13
created March 24th, 2018
by Nina Oghoore, Kaiyi and Asmaa
*/
#include "SD.h"
#define SD_ChipSelectPin 10
#include "TMRpcm.h"
#include "SPI.h"
TMRpcm tunes;
boolean playingMusic1=false; //can either be true (audio is playing) or false (audio is not playing)
boolean playingMusic2=false; //can either be true (audio is playing) or false (audio is not playing)
boolean playingMusic3=false; //can either be true (audio is playing) or false (audio is not playing)
boolean playingMusic4=false; //can either be true (audio is playing) or false (audio is not playing)
void setup(){
tunes.speakerPin = 9;
Serial.begin(9600);
if (!SD.begin(SD_ChipSelectPin)) {
Serial.println("SD fail");
return;
}
pinMode(2,INPUT); //PushButton1 to play track
pinMode(3,INPUT); //PushButton2 to play track
pinMode(4,INPUT); //PushButton3 to play track
pinMode(5,INPUT); //PushButton4 to play track
pinMode(9,OUTPUT); //Speaker pin
tunes.setVolume(5); //Volume range between 0 and 7
//tunes.quality(0);
}
void loop() {
Push1(); //Pulls and loops function below
Push2(); //Pulls and loops function below
Push3(); //Pulls and loops function below
Push4(); //Pulls and loops function below
}
void Push1() {
if (digitalRead(2)==LOW && playingMusic1==false) //PushButton1 pressed and audio is off
{
playingMusic1=true; //turn on audio
Serial.println("push1 detected"); //print to serial monitor
tunes.play("0.wav",33); //play track from the 33rd second
}
if (digitalRead(2)==HIGH && playingMusic1==true) //PushButton1 not pressed and audio is on
{
tunes.pause(); //turn off audio
playingMusic1=false; //audio is off
}
}
void Push2() {
if (digitalRead(3)==LOW && playingMusic2==false) //PushButton2 pressed and audio is off
{
playingMusic2=true; //turn on audio
Serial.println("push2 detected"); //print to serial monitor
tunes.play("0.wav",120); //play track from the 120th second
}
if (digitalRead(3)==HIGH && playingMusic2==true) //PushButton2 not pressed and audio is on
{
tunes.pause(); //turn off audio
playingMusic2=false; //audio is off
}
}
void Push3() {
if (digitalRead(4)==LOW && playingMusic3==false) //PushButton3 pressed and audio is off
{
playingMusic3=true; //turn on audio
Serial.println("push3 detected"); //print to serial monitor
tunes.play("0.wav",59); //play track from the 59th second
}
if (digitalRead(4)==HIGH && playingMusic3==true) //PushButton3 not pressed and audio is on
{
tunes.pause(); //turn off audio
playingMusic3=false; //audio is off
}
}
void Push4() {
if (digitalRead(5)==LOW && playingMusic4==false) //PushButton4 pressed and audio is off
{
playingMusic4=true; //turn on audio
Serial.println("push4 detected"); //print to serial monitor
tunes.play("0.wav",20); //play track from the 20th second
}
if (digitalRead(5)==HIGH && playingMusic4==true) //PushButton4 not pressed and audio is on
{
tunes.pause(); //turn off audio
playingMusic4=false; //audio is off
}
}
Note: For this project, we chose to use one audio file and have the different push buttons set up to play from different points in the audio file, by adding the time in seconds below to tell Arduino to play from that point (time in the track).
For example:
tunes.play("0.wav",20); //play track from the 20th second
The video below shows the project in action and explains how it works. It is really simple to operate, you push any of the four push buttons and it plays the audio.
Created by: Nina Oghoore, Kaiyi Li, Asmaa Morsi Ellithy
Comments
Post a Comment